Ternary search
Hi! This time I going to talk about a new technique I’ve just learned, Ternary Search.
Ternary search
Ternary search is a technique similar to Binary Search and is important since ternary search could success in situations where the binary search would fail. Time ago I was very criticized in the Codeforces forum for arguing that has no sense to use ternary search if it is not more efficient than binary search. Is not about efficiency but –said the others. Actually I meant to ternary search in arrays, to reduce the search space to a third part, etc. Now I understand what they were talking about.
Sample problem
You can solve the 1146 - Closest Distance problem from lightoj.com with this technique.
Solution to sample problem
Basic idea
Compute the distance between the two men as they move and take the minimum distance.
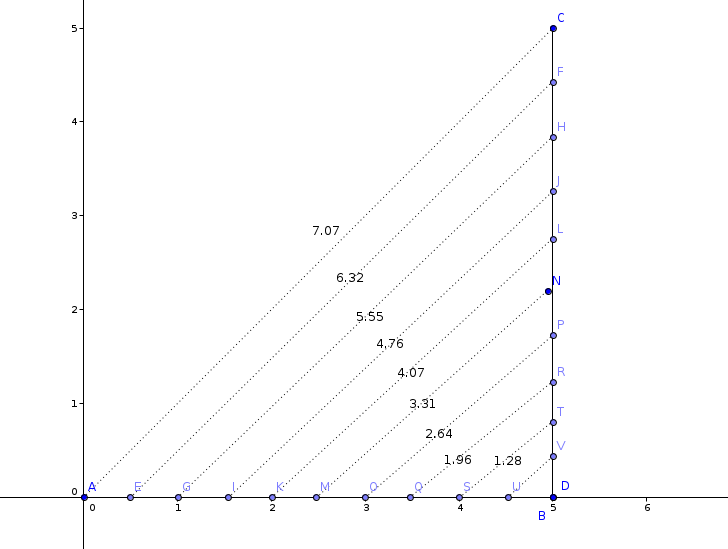
Obviously, we can only take discrete points, and, since the problem ask for an answer with an error less than 1e-6, a brute force approach would take much time, very much. So the strategy is to use ternary search:
And so on. Here is my solution:
#include <iostream>
#include <cstdio>
#include <cmath>
#include <complex>
#include <algorithm>
#define x() real()
#define y() imag()
using namespace std;
typedef complex<double> Vector;
typedef complex<double> point;
5];
point points[
double closest()
{1] - points[0];
Vector A = points[3] - points[2];
Vector B = points[
Vector unita = A/abs(A);
Vector unitb = B/abs(B);0];
point startA = points[2];
point startB = points[
double maxa = abs(A);
double mina = 0;
double maxb = abs(B);
double minb = 0;
double ta, tb, ans = 1000;
point a, b, c, d;
int count = 500;
while (count--) {
3;
ta = abs(maxa - mina)/3;
tb = abs(maxb - minb)/
a = (unita * (mina + ta)) + startA;
b = (unitb * (minb + tb)) + startB;
ta += ta;
tb += tb;
c = (unita * (mina + ta)) + startA;
d = (unitb * (minb + tb)) + startB;
if (abs(b - a) < abs(d - c)) {
2;
maxa = maxa - ta/2;
maxb = maxb - tb/std::min(ans, abs(b - a));
ans = else {
} 2;
mina = mina + ta/2;
minb = minb + tb/std::min(ans, abs(d - c));
ans =
}
}
return ans;
}
int main(int argc, char **argv)
{int t, tc, i;
double x, y;
"%d", &t);
scanf(
for (tc = 1; tc <= t; tc++) {
for (i = 0; i < 4; i++) {
"%lf %lf", &x, &y);
scanf(
points[i] = point(x, y);
}
"Case %d: %.10lf\n", tc, closest());
printf(
}
return 0;
}
References
1 | Ternary search |
2 | Codeforces discussion about ternary search |
3 | Topcoder discussion about ternary search |